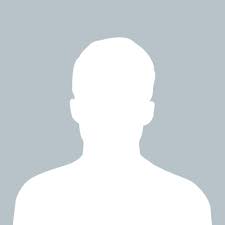
0
Margarett posted
Android - FCM give success but Notification is not received by device in Android
<service
android:name=".fcm.MyFirebaseMessagingService"
android:permission="com.google.android.c2dm.permission.SEND">
<intent-filter>
<action android:name="com.google.firebase.MESSAGING_EVENT" />
<action android:name="com.google.android.c2dm.intent.RECEIVE" />
</intent-filter>
</service>
package com.example.fcm;
import android.app.NotificationManager;
import android.app.PendingIntent;
import android.content.Context;
import android.content.Intent;
import android.media.RingtoneManager;
import android.net.Uri;
import android.util.Log;
import androidx.core.app.NotificationCompat;
import com.google.firebase.messaging.FirebaseMessagingService;
import com.google.firebase.messaging.RemoteMessage;
import com.wholesaleveggy.ui.SigninActivity;
/**
* Created by archirayan on 29/5/19.
Author:www.archirayan.com
Email:info@archirayan.com
*/
public class MyFirebaseMessagingService extends FirebaseMessagingService {
private static final String TAG = MyFirebaseMessagingService.class.getName();
private Context context;
private String event_title, event_date;
@Override
public void onMessageReceived(RemoteMessage remoteMessage) {
context = MyFirebaseMessagingService.this.getApplicationContext();
Log.e(TAG, "From: " + remoteMessage.getFrom());
if (remoteMessage.getData().size() > 0) {
event_title = remoteMessage.getData().get("push_title");
event_date = remoteMessage.getData().get("push_message");
sendNotification(event_title);
}
if (remoteMessage.getNotification() != null) {
Log.d(TAG, "Message Notification Body:" + remoteMessage.getNotification().getBody());
sendNotification(remoteMessage.getNotification().getBody());
}
}
private void sendNotification(String str) {
Intent intent = new Intent(context, SigninActivity.class);
intent.addFlags(Intent.FLAG_ACTIVITY_CLEAR_TOP);
PendingIntent pendingIntent = PendingIntent.getActivity(context, 0, intent,
PendingIntent.FLAG_CANCEL_CURRENT);
Uri defaultSoundUri = RingtoneManager.getDefaultUri(RingtoneManager.TYPE_NOTIFICATION);
NotificationCompat.Builder notificationBuilder = new NotificationCompat.Builder(context)
.setContentTitle("WV")
.setContentText(str)
//.addAction(new NotificationCompat.Action(R.mipmap.ic_launcher_round, "Accept", pendingIntent))
//.addAction(new NotificationCompat.Action(R.mipmap.ic_launcher_round, "Cancle", pendingIntent))
.setAutoCancel(true)
//.setStyle(new NotificationCompat.BigPictureStyle().bigPicture(bitmap))
.setSound(defaultSoundUri)
.setContentIntent(pendingIntent);
NotificationManager notificationManager =
(NotificationManager) context.getSystemService(Context.NOTIFICATION_SERVICE);
notificationManager.notify(0, notificationBuilder.build());
}
}
i am not getting notification at android device with given device token